<자바빈즈>
1. 좁은 의미: (자바에)데이터 저장 클래스
-> 접근제한자 private(은닉화, bean을 보호), setter, getter
2. 넓은 의미: java로 만든 프로그램
=> 1을 jsp에서 사용하게 해주는 액션 태그
-용도
1)jsp 페이지가 복잡한 자바코드로 구성되는 것을 가능한 피하고, 쉽고 간단한 코드로 구성하도록 한다.
-> 가독성 up
-> 단, 액션 태그는 "서버"에서 해석된다(웹 브라우저X)
2)전달 객체로 사용(언제든 재사용 가능)
-> 많은 데이터를 하나로 포장해 안전하게 던지는 방법
-> 포장들이 많은 경우: Vector(thread safe)/ArrayList(thread safeX) like 이중포
1. 빈 클래스 생성
: 변수, setter, getter
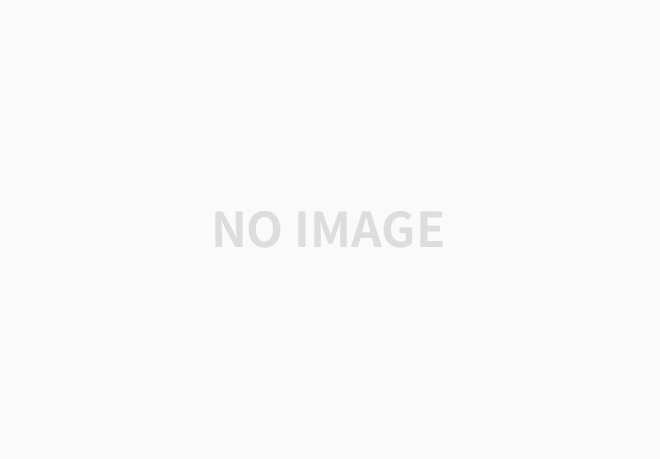
변수 작성
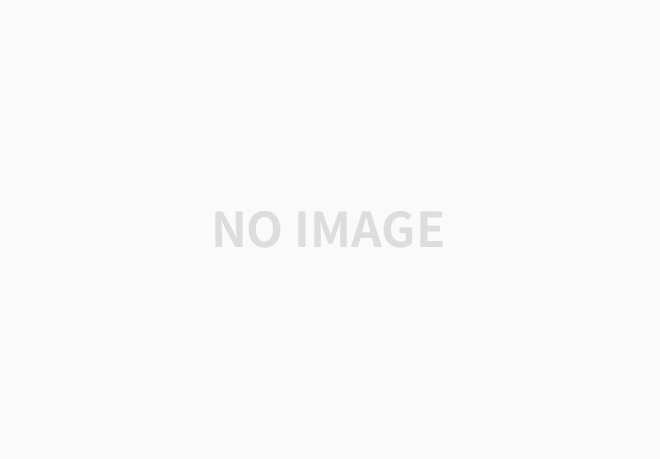
setter, getter 클래스 작성
-> 손코딩 금지(버그 못 찾는다)
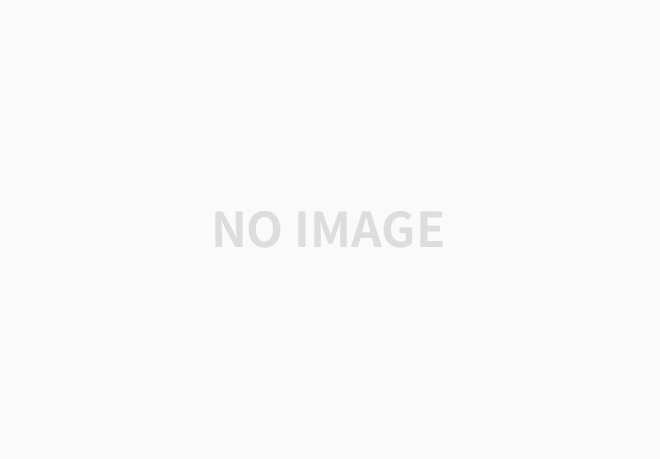
source-getters and setters를 통해 클래스 생성
2. jsp파일 생성
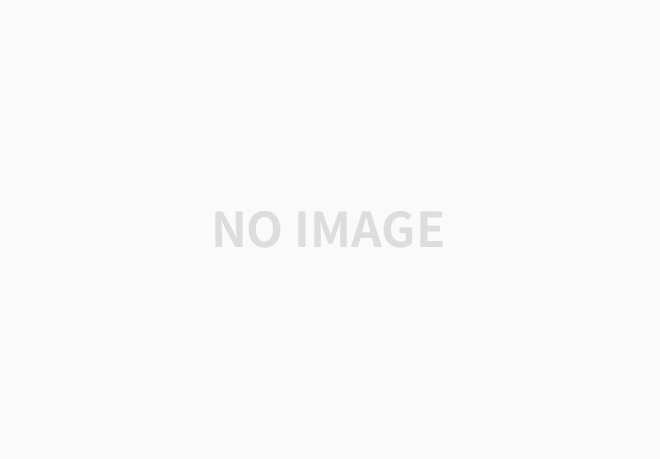
useBean 액션의 가장 기본적인 속성 요소
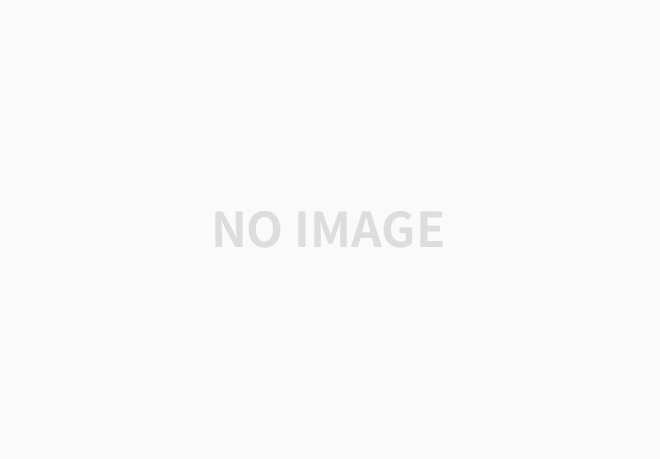
setter, getter 액션태그 작성
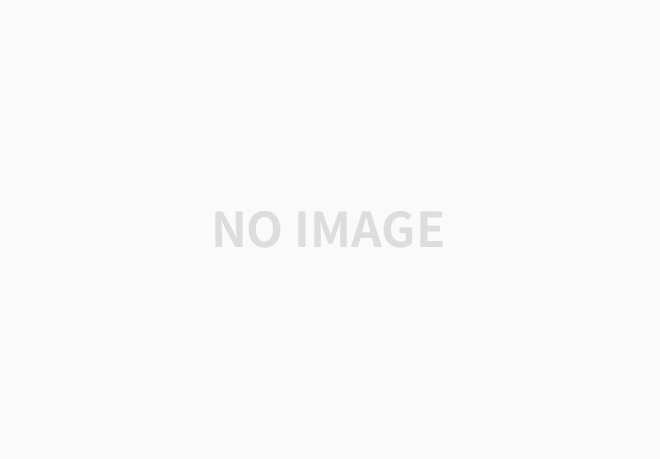
결과값
<빈을 이용한 회원가입 양식>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
|
<title>회원가입</title>
<!-- <link href="style.css" rel="stylesheet" type="text/css">
<script language="JavaScript" src="script.js"></script> -->
</head>
<body bgcolor = "#996600">
<table width = "80%" align = "center" border = "0" cellspacin = "0" cellpadding = "5">
<tr>
<td align = "center" valign = "middle" bgcolor = "#FFFFCC">
<table width = "90%" border = "1" cellspacing = "0" cellpadding = "2" align = "center">
<tr align = "center" bgcolor = "#996600">
<td colspan = "3"><font color = "#FFFFFF"><b>회원 가입</b></font></td>
<tr/>
<tr>
<td width = "100">아이디</td>
<td width = "200"><input name = "id" size = "15"></td>
<td width = "200">아이디를 입력하세요.</td>
</tr>
<tr>
<td>패스워드</td>
<td><input type = "password" name = "pwd" size = "15"></td>
<td>패스워드를 입력하세요.</td>
</tr>
<tr>
<td>패스워드 확인</td>
<td><input type = "password" name = "repwd" size = "15"></td>
<td>패스워드를 확인합니다.</td>
</tr>
<tr>
<td>이름</td>
<td><input name = "name" size = "15"></td>
<td>고객 실명을 입력하세요.</td>
</tr>
<tr>
<td>생년월일</td>
<td><input name = "birthday" size = "27"></td>
<td>생년월일을 입력하세요.</td>
</tr>
<tr>
<td>이메일</td>
<td><input name = "email" size = "20"></td>
<td>이메일을 입력하세요.</td>
</tr>
<tr>
<td colspan = "3" align = "center">
<input type = "submit" value = "회원가입"><!-- onclick = "inputCheck()" -->
<input type = "reset" value = "다시쓰기">
</td>
</tr>
</form>
</table>
</td>
</tr>
</body>
</html>
http://colorscripter.com/info#e" target="_blank" style="color:#e5e5e5text-decoration:none">Colored by Color Scripter
|
회원가입 입력 폼
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
|
public class MemberBean {
private String id;
private String pwd;
private String name;
private String birthday;
private String email;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getPwd() {
return pwd;
}
public void setPwd(String pwd) {
this.pwd = pwd;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getBirthday() {
return birthday;
}
public void setBirthday(String birthday) {
this.birthday = birthday;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
http://colorscripter.com/info#e" target="_blank" style="color:#e5e5e5text-decoration:none">Colored by Color Scripter
|
회원 정보 처리 부분(자바 빈)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
|
<title>회원가입 확인</title>
<!-- <link href = "style.css" rel = "stylesheet" type = "text/css"> -->
</head>
<body bgcolor = "#996600">
<table width = "80%" align = "center" border = "0" cellspacing = "0" cellpadding = "5">
<tr>
<td = align = "center" valign = "middle" bgcolor = "#FFFFCC">
<table width = "90%" border = "1" cellspacing = "0" cellpadding = "2" align = "center">
<tr align = "center" bgcolor = "#996600">
<td colspan = "3"><font color = "#996600"><b>
<jsp:getProperty property="name" name="regBean"/>
회원님이 작성하신 내용입니다. 확인해 주세요.
</b></font>
</td>
</tr>
<tr>
<td width = "24%">아이디</td>
<td width = "41%"><jsp:getProperty property="id" name="regBean"/></td>
</tr>
<tr>
<td>비밀번호</td>
<td><jsp:getProperty property="pwd" name="regBean"/></td>
</tr>
<tr>
<td>이름</td>
<td><jsp:getProperty property="name" name="regBean"/></td>
</tr>
<tr>
<td>생년월일</td>
<td><jsp:getProperty property="birthday" name="regBean"/></td>
</tr>
<tr>
<td>이메일</td>
<td><jsp:getProperty property="email" name="regBean"/></td>
</tr>
<tr>
<td colspan = "3" align = "center">
<input type = "button" value = "확인완료">
</td>
</tr>
</form>
</table>
</td>
</tr>
</table>
</body>
</html>
http://colorscripter.com/info#e" target="_blank" style="color:#e5e5e5text-decoration:none">Colored by Color Scripter
|
회원입력 정보확인 페이지
*아직 memberInsert 페이지는 구현하지 않았다.
-실행
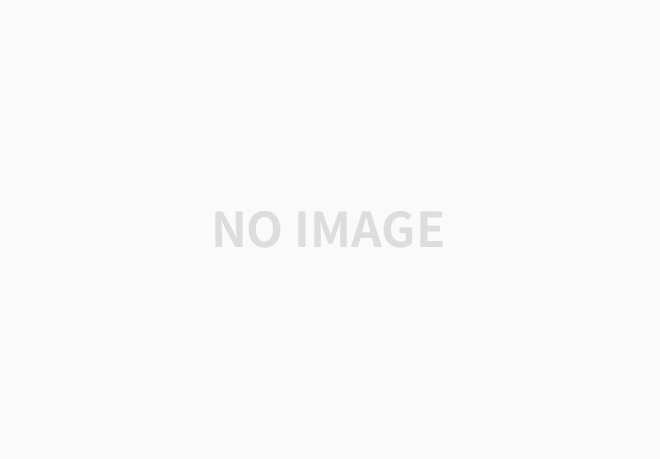
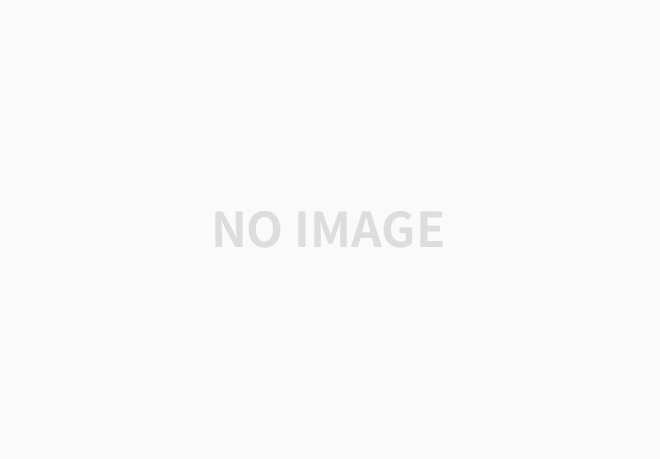
'20.03 ~ 20.08 국비교육 > JSP' 카테고리의 다른 글
데이터베이스 기본 개념 (0) | 2020.04.28 |
---|---|
데이터베이스(개발환경 구축 및 기본 MySQL 구문) (0) | 2020.04.27 |
내부객체3. page, config, exception (0) | 2020.04.24 |
내부객체2. session, application, pageContext (0) | 2020.04.24 |
내부객체1. request, response, out (0) | 2020.04.23 |